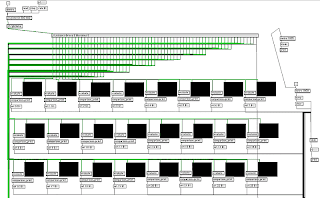
I'm using max/msp/jitter to evaluate the video stream. the code is somewhat bulky and there might be potential ways to streamline, but it is functional. I essentially took the stream, converted into a single luminance plane and cut up into 64 matrix segments with the scissor object. Each matrix, which correspond to an individual physical cube, is evaluated to determine a luminance mean value between 0-255, black to white. This average value is then evaluated on a simple 3 part scale. Numbers ranging from 0-84 are considered black, 85-166 is gray and 167-255 is white. Whatever segment comes back true is given another value; either a 0, 1 or 2 and packed into an indexed table. This table with all the associated values are sent though serial to the basic stamp. For now, this is done through a metro that alternatively dumps the table and then clears it in order to receive the new set of 64. I plan to try a funbuff object as well, to see if this is easier to serial the message to the basic stamp, without the need of arbitrary timing and memory clearing.
I've included the code for those with max who may want to see the program at work. There are two separate objects included which perform both sets of evaluations.
Mean Luminance Value:
evaluate
max v2;
#N vpatcher 10 59 610 459;
#P outlet 189 149 15 0;
#P inlet 189 35 15 0;
#P window setfont "Sans Serif" 9.;
#P window linecount 1;
#P newex 189 111 40 196617 unpack;
#P newex 188 70 53 196617 jit.3m;
#P connect 2 0 0 0;
#P fasten 0 1 1 0 207 100 194 100;
#P connect 1 0 3 0;
#P pop;
Scale Comparison:
comparison print
max v2;
#N vpatcher 285 266 936 837;
#P outlet 274 353 15 0;
#P window setfont "Sans Serif" 9.;
#P number 274 321 35 9 0 0 0 3 0 0 0 221 221 221 222 222 222 0 0 0;
#P window linecount 1;
#P message 351 223 14 196617 3;
#P message 278 261 14 196617 2;
#P message 143 216 14 196617 1;
#P inlet 283 44 15 0;
#P newex 354 176 46 196617 select 1;
#P newex 353 125 42 196617 >= 167;
#P newex 143 177 46 196617 select 1;
#P number 353 151 35 9 0 0 0 3 0 0 0 221 221 221 222 222 222 0 0 0;
#P number 143 152 35 9 0 0 0 3 0 0 0 221 221 221 222 222 222 0 0 0;
#P newex 143 126 36 196617 <= 84; #P newex 277 226 46 196617 select 1; #P number 277 204 35 9 0 0 0 3 0 0 0 221 221 221 222 222 222 0 0 0; #P number 293 151 35 9 0 0 0 3 0 0 0 221 221 221 222 222 222 0 0 0; #P newex 277 180 27 196617 &&amp;amp;amp;; #P number 251 151 35 9 0 0 0 3 0 0 0 221 221 221 222 222 222 0 0 0; #P newex 293 125 42 196617 <= 166; #P newex 251 125 36 196617 >= 85;
#P number 283 81 35 9 0 0 0 3 0 0 0 221 221 221 222 222 222 0 0 0;
#P fasten 0 0 8 0 288 108 146 108 146 128 148 127;
#P connect 8 0 9 0;
#P connect 9 0 11 0;
#P connect 11 0 15 0;
#P fasten 0 0 1 0 288 109 252 109 256 126;
#P connect 1 0 3 0;
#P fasten 17 0 18 0 356 305 284 305 284 309 279 309;
#P fasten 16 0 18 0 283 308 279 308;
#P fasten 15 0 18 0 148 308 279 308;
#P connect 18 0 19 0;
#P fasten 3 0 4 0 256 174 281 174 281 183 282 182;
#P connect 4 0 6 0;
#P connect 6 0 7 0;
#P connect 7 0 16 0;
#P connect 14 0 0 0;
#P fasten 0 0 2 0 288 109 298 109;
#P connect 2 0 5 0;
#P connect 5 0 4 1;
#P connect 13 0 17 0;
#P fasten 0 0 12 0 288 108 358 108;
#P connect 12 0 10 0;
#P connect 10 0 13 0;
#P pop;
Video Matrix Scissor:
max v2;
#N vpatcher 38 70 1375 1222;
#P origin 85 3;
#P window setfont "Sans Serif" 9.;
#P message 1158 194 35 196617 break;
#P newex 1157 216 32 196617 print;
#P newex 1157 169 64 196617 metro 1005;
#P newex 1283 665 32 196617 print;
#P number 1280 624 35 9 0 0 0 3 0 0 0 221 221 221 222 222 222 0 0 0;
#P toggle 1216 356 15 0;
#P newex 1218 383 64 196617 metro 1000;
#P message 1219 412 33 196617 dump;
#P message 1220 437 33 196617 clear;
#P window linecount 0;
#P newex 1189 1606 50 196617;
#P window linecount 1;
#N vtable 64 20 74 230 241 9700 3;
#P newobj 1279 584 32 196617 table;
#P message 1009 1557 54 196617 set 63 \$1;
#P newex 1009 1538 89 196617 comparison_print;
#P newex 1010 1512 48 196617 evaluate;
#P message 873 1557 54 196617 set 62 \$1;
#P newex 873 1538 89 196617 comparison_print;
#P newex 874 1512 48 196617 evaluate;
#P message 740 1556 54 196617 set 61 \$1;
#P newex 739 1537 89 196617 comparison_print;
#P newex 740 1511 48 196617 evaluate;
#P message 604 1557 54 196617 set 60 \$1;
#P newex 604 1538 89 196617 comparison_print;
#P newex 605 1512 48 196617 evaluate;
#P message 468 1559 54 196617 set 59 \$1;
#P newex 468 1540 89 196617 comparison_print;
#P newex 469 1514 48 196617 evaluate;
#P message 333 1559 54 196617 set 58 \$1;
#P newex 332 1540 89 196617 comparison_print;
#P newex 333 1514 48 196617 evaluate;
#P message 193 1561 54 196617 set 57 \$1;
#P newex 193 1541 89 196617 comparison_print;
#P newex 194 1515 48 196617 evaluate;
#P message 55 1563 54 196617 set 56 \$1;
#P newex 54 1542 89 196617 comparison_print;
#P newex 55 1516 48 196617 evaluate;
#P user jit.pwindow 1066 1474 82 62 0 1 0 0 1 0;
#P user jit.pwindow 925 1475 82 62 0 1 0 0 1 0;
#P user jit.pwindow 790 1475 82 62 0 1 0 0 1 0;
#P user jit.pwindow 655 1475 82 62 0 1 0 0 1 0;
#P user jit.pwindow 520 1476 82 62 0 1 0 0 1 0;
#P user jit.pwindow 383 1476 82 62 0 1 0 0 1 0;
#P user jit.pwindow 246 1474 82 62 0 1 0 0 1 0;
#P user jit.pwindow 104 1474 82 62 0 1 0 0 1 0;
#P message 1011 1424 54 196617 set 55 \$1;
#P newex 1011 1397 89 196617 comparison_print;
#P newex 1012 1371 48 196617 evaluate;
#P message 875 1424 54 196617 set 54 \$1;
#P newex 875 1397 89 196617 comparison_print;
#P newex 876 1371 48 196617 evaluate;
#P message 741 1423 54 196617 set 53 \$1;
#P newex 741 1396 89 196617 comparison_print;
#P newex 742 1370 48 196617 evaluate;
#P message 606 1424 54 196617 set 52 \$1;
#P newex 606 1397 89 196617 comparison_print;
#P newex 607 1371 48 196617 evaluate;
#P message 470 1426 54 196617 set 51 \$1;
#P newex 470 1399 89 196617 comparison_print;
#P newex 471 1373 48 196617 evaluate;
#P message 335 1426 54 196617 set 50 \$1;
#P newex 334 1399 89 196617 comparison_print;
#P newex 335 1373 48 196617 evaluate;
#P message 196 1427 54 196617 set 49 \$1;
#P newex 195 1400 89 196617 comparison_print;
#P newex 196 1374 48 196617 evaluate;
#P message 57 1428 54 196617 set 48 \$1;
#P newex 56 1401 89 196617 comparison_print;
#P newex 57 1375 48 196617 evaluate;
#P user jit.pwindow 1061 1333 82 62 0 1 0 0 1 0;
#P user jit.pwindow 927 1334 82 62 0 1 0 0 1 0;
#P user jit.pwindow 792 1334 82 62 0 1 0 0 1 0;
#P user jit.pwindow 657 1334 82 62 0 1 0 0 1 0;
#P user jit.pwindow 522 1335 82 62 0 1 0 0 1 0;
#P user jit.pwindow 385 1335 82 62 0 1 0 0 1 0;
#P user jit.pwindow 248 1333 82 62 0 1 0 0 1 0;
#P user jit.pwindow 106 1333 82 62 0 1 0 0 1 0;
#P message 1017 1262 54 196617 set 47 \$1;
#P newex 1017 1235 89 196617 comparison_print;
#P newex 1018 1209 48 196617 evaluate;
#P message 881 1262 54 196617 set 46 \$1;
#P newex 881 1235 89 196617 comparison_print;
#P newex 882 1209 48 196617 evaluate;
#P message 747 1261 54 196617 set 45 \$1;
#P newex 747 1234 89 196617 comparison_print;
#P newex 748 1208 48 196617 evaluate;
#P message 612 1262 54 196617 set 44 \$1;
#P newex 612 1235 89 196617 comparison_print;
#P newex 613 1209 48 196617 evaluate;
#P message 476 1264 54 196617 set 43 \$1;
#P newex 476 1237 89 196617 comparison_print;
#P newex 477 1211 48 196617 evaluate;
#P message 341 1264 54 196617 set 42 \$1;
#P newex 340 1237 89 196617 comparison_print;
#P newex 341 1211 48 196617 evaluate;
#P message 202 1265 54 196617 set 41 \$1;
#P newex 201 1238 89 196617 comparison_print;
#P newex 202 1212 48 196617 evaluate;
#P message 63 1266 54 196617 set 40 \$1;
#P newex 62 1239 89 196617 comparison_print;
#P newex 63 1213 48 196617 evaluate;
#P user jit.pwindow 1067 1171 82 62 0 1 0 0 1 0;
#P user jit.pwindow 933 1172 82 62 0 1 0 0 1 0;
#P user jit.pwindow 798 1172 82 62 0 1 0 0 1 0;
#P user jit.pwindow 663 1172 82 62 0 1 0 0 1 0;
#P user jit.pwindow 528 1173 82 62 0 1 0 0 1 0;
#P user jit.pwindow 391 1173 82 62 0 1 0 0 1 0;
#P user jit.pwindow 254 1171 82 62 0 1 0 0 1 0;
#P user jit.pwindow 112 1171 82 62 0 1 0 0 1 0;
#P message 1029 1107 54 196617 set 39 \$1;
#P newex 1029 1080 89 196617 comparison_print;
#P newex 1030 1054 48 196617 evaluate;
#P message 893 1107 54 196617 set 38 \$1;
#P newex 893 1080 89 196617 comparison_print;
#P newex 894 1054 48 196617 evaluate;
#P message 759 1106 54 196617 set 37 \$1;
#P newex 759 1079 89 196617 comparison_print;
#P newex 760 1053 48 196617 evaluate;
#P message 627 1107 54 196617 set 36 \$1;
#P newex 624 1080 89 196617 comparison_print;
#P newex 625 1054 48 196617 evaluate;
#P message 488 1109 54 196617 set 35 \$1;
#P newex 488 1082 89 196617 comparison_print;
#P newex 489 1056 48 196617 evaluate;
#P message 353 1109 54 196617 set 34 \$1;
#P newex 352 1082 89 196617 comparison_print;
#P newex 353 1056 48 196617 evaluate;
#P message 213 1111 54 196617 set 33 \$1;
#P newex 213 1083 89 196617 comparison_print;
#P newex 214 1057 48 196617 evaluate;
#P message 77 1114 54 196617 set 32 \$1;
#P newex 74 1084 89 196617 comparison_print;
#P newex 75 1058 48 196617 evaluate;
#P user jit.pwindow 1079 1016 82 62 0 1 0 0 1 0;
#P user jit.pwindow 945 1017 82 62 0 1 0 0 1 0;
#P user jit.pwindow 810 1017 82 62 0 1 0 0 1 0;
#P user jit.pwindow 675 1017 82 62 0 1 0 0 1 0;
#P user jit.pwindow 540 1018 82 62 0 1 0 0 1 0;
#P user jit.pwindow 403 1018 82 62 0 1 0 0 1 0;
#P user jit.pwindow 266 1016 82 62 0 1 0 0 1 0;
#P user jit.pwindow 124 1016 82 62 0 1 0 0 1 0;
#P message 1014 968 54 196617 set 31 \$1;
#P newex 1014 941 89 196617 comparison_print;
#P newex 1015 915 48 196617 evaluate;
#P message 878 968 54 196617 set 30 \$1;
#P newex 878 941 89 196617 comparison_print;
#P newex 879 915 48 196617 evaluate;
#P message 744 967 54 196617 set 29 \$1;
#P newex 744 940 89 196617 comparison_print;
#P newex 745 914 48 196617 evaluate;
#P message 609 968 54 196617 set 28 \$1;
#P newex 609 941 89 196617 comparison_print;
#P newex 610 915 48 196617 evaluate;
#P message 473 970 54 196617 set 27 \$1;
#P newex 473 943 89 196617 comparison_print;
#P newex 474 917 48 196617 evaluate;
#P message 338 970 54 196617 set 26 \$1;
#P newex 337 943 89 196617 comparison_print;
#P newex 338 917 48 196617 evaluate;
#P message 199 971 54 196617 set 25 \$1;
#P newex 198 944 89 196617 comparison_print;
#P newex 199 918 48 196617 evaluate;
#P message 60 972 54 196617 set 24 \$1;
#P newex 59 945 89 196617 comparison_print;
#P newex 60 919 48 196617 evaluate;
#P user jit.pwindow 1064 877 82 62 0 1 0 0 1 0;
#P user jit.pwindow 930 878 82 62 0 1 0 0 1 0;
#P user jit.pwindow 795 878 82 62 0 1 0 0 1 0;
#P user jit.pwindow 660 878 82 62 0 1 0 0 1 0;
#P user jit.pwindow 525 879 82 62 0 1 0 0 1 0;
#P user jit.pwindow 388 879 82 62 0 1 0 0 1 0;
#P user jit.pwindow 251 877 82 62 0 1 0 0 1 0;
#P user jit.pwindow 109 877 82 62 0 1 0 0 1 0;
#P message 1017 772 54 196617 set 23 \$1;
#P newex 1017 745 89 196617 comparison_print;
#P newex 1018 719 48 196617 evaluate;
#P message 881 772 54 196617 set 22 \$1;
#P newex 881 745 89 196617 comparison_print;
#P newex 882 719 48 196617 evaluate;
#P message 747 771 54 196617 set 21 \$1;
#P newex 747 744 89 196617 comparison_print;
#P newex 748 718 48 196617 evaluate;
#P message 612 772 54 196617 set 20 \$1;
#P newex 612 745 89 196617 comparison_print;
#P newex 613 719 48 196617 evaluate;
#P message 476 774 54 196617 set 19 \$1;
#P newex 476 747 89 196617 comparison_print;
#P newex 477 721 48 196617 evaluate;
#P message 341 774 54 196617 set 18 \$1;
#P newex 340 747 89 196617 comparison_print;
#P newex 341 721 48 196617 evaluate;
#P message 202 775 54 196617 set 17 \$1;
#P newex 201 748 89 196617 comparison_print;
#P newex 202 722 48 196617 evaluate;
#P message 63 776 54 196617 set 16 \$1;
#P newex 62 749 89 196617 comparison_print;
#P newex 63 723 48 196617 evaluate;
#P user jit.pwindow 1067 681 82 62 0 1 0 0 1 0;
#P user jit.pwindow 933 682 82 62 0 1 0 0 1 0;
#P user jit.pwindow 798 682 82 62 0 1 0 0 1 0;
#P user jit.pwindow 663 682 82 62 0 1 0 0 1 0;
#P user jit.pwindow 528 683 82 62 0 1 0 0 1 0;
#P user jit.pwindow 391 683 82 62 0 1 0 0 1 0;
#P user jit.pwindow 254 681 82 62 0 1 0 0 1 0;
#P user jit.pwindow 112 681 82 62 0 1 0 0 1 0;
#P message 1021 614 54 196617 set 15 \$1;
#P newex 1021 587 89 196617 comparison_print;
#P newex 1022 561 48 196617 evaluate;
#P message 885 614 54 196617 set 14 \$1;
#P newex 885 587 89 196617 comparison_print;
#P newex 886 561 48 196617 evaluate;
#P message 751 613 54 196617 set 13 \$1;
#P newex 751 586 89 196617 comparison_print;
#P newex 752 560 48 196617 evaluate;
#P message 616 614 54 196617 set 12 \$1;
#P newex 616 587 89 196617 comparison_print;
#P newex 617 561 48 196617 evaluate;
#P message 481 613 54 196617 set 11 \$1;
#P newex 480 589 89 196617 comparison_print;
#P newex 481 563 48 196617 evaluate;
#P message 345 616 54 196617 set 10 \$1;
#P newex 344 589 89 196617 comparison_print;
#P newex 345 563 48 196617 evaluate;
#P message 206 617 48 196617 set 9 \$1;
#P newex 205 590 89 196617 comparison_print;
#P newex 206 564 48 196617 evaluate;
#P message 67 617 48 196617 set 8 \$1;
#P newex 66 591 89 196617 comparison_print;
#P newex 67 565 48 196617 evaluate;
#P user jit.pwindow 1071 523 82 62 0 1 0 0 1 0;
#P user jit.pwindow 937 524 82 62 0 1 0 0 1 0;
#P user jit.pwindow 802 524 82 62 0 1 0 0 1 0;
#P user jit.pwindow 667 524 82 62 0 1 0 0 1 0;
#P user jit.pwindow 532 525 82 62 0 1 0 0 1 0;
#P user jit.pwindow 395 525 82 62 0 1 0 0 1 0;
#P user jit.pwindow 258 523 82 62 0 1 0 0 1 0;
#P user jit.pwindow 116 523 82 62 0 1 0 0 1 0;
#P message 1010 444 48 196617 set 7 \$1;
#P newex 1010 417 89 196617 comparison_print;
#P newex 1011 391 48 196617 evaluate;
#P message 874 444 48 196617 set 6 \$1;
#P newex 874 417 89 196617 comparison_print;
#P newex 875 391 48 196617 evaluate;
#P message 740 443 48 196617 set 5 \$1;
#P newex 740 416 89 196617 comparison_print;
#P newex 741 390 48 196617 evaluate;
#P message 605 444 48 196617 set 4 \$1;
#P newex 605 417 89 196617 comparison_print;
#P newex 606 391 48 196617 evaluate;
#P message 469 446 48 196617 set 3 \$1;
#P newex 469 419 89 196617 comparison_print;
#P newex 470 393 48 196617 evaluate;
#P message 334 446 48 196617 set 2 \$1;
#P newex 333 419 89 196617 comparison_print;
#P newex 334 393 48 196617 evaluate;
#P message 195 447 48 196617 set 1 \$1;
#P newex 194 420 89 196617 comparison_print;
#P newex 195 394 48 196617 evaluate;
#P message 56 448 48 196617 set 0 \$1;
#P newex 55 421 89 196617 comparison_print;
#P newex 56 395 48 196617 evaluate;
#P newex 41 119 66 196617 jit.rgb2luma;
#P user jit.pwindow 1060 353 82 62 0 1 0 0 1 0;
#P user jit.pwindow 926 354 82 62 0 1 0 0 1 0;
#P user jit.pwindow 791 354 82 62 0 1 0 0 1 0;
#P user jit.pwindow 656 354 82 62 0 1 0 0 1 0;
#P user jit.pwindow 521 355 82 62 0 1 0 0 1 0;
#P user jit.pwindow 384 355 82 62 0 1 0 0 1 0;
#P user jit.pwindow 247 353 82 62 0 1 0 0 1 0;
#P user jit.pwindow 105 353 82 62 0 1 0 0 1 0;
#P newex 224 157 846 196617 jit.scissors @rows 8 @columns 8;
#P newex 37 51 42 196617 qmetro;
#P message 136 47 28 196617 stop;
#P flonum 168 22 35 9 0 0 0 3 0 0 0 221 221 221 222 222 222 0 0 0;
#P message 168 47 42 196617 rate \$1;
#P message 105 47 28 196617 read;
#P toggle 40 26 15 0;
#P newex 40 85 105 196617 jit.qt.movie 600 600;
#P connect 1 0 6 0;
#P connect 6 0 0 0;
#P fasten 5 0 0 0 141 73 45 73;
#P fasten 3 0 0 0 173 73 45 73;
#P fasten 2 0 0 0 110 73 45 73;
#P connect 0 0 16 0;
#P connect 241 0 242 0;
#P connect 17 0 18 0;
#P fasten 7 57 241 0 970 196 45 196 51 1075 60 1075;
#P connect 242 0 243 0;
#P fasten 7 0 17 0 229 338 61 338;
#P connect 18 0 19 0;
#P connect 209 0 210 0;
#P fasten 7 49 209 0 866 215 45 215 45 968 62 968;
#P connect 210 0 211 0;
#P connect 113 0 114 0;
#P fasten 7 25 113 0 554 281 45 281 45 838 65 838;
#P connect 114 0 115 0;
#P connect 81 0 82 0;
#P connect 177 0 178 0;
#P fasten 7 17 81 0 450 305 43 305 43 667 68 667;
#P connect 82 0 83 0;
#P fasten 7 41 177 0 762 240 48 240 48 1159 68 1159;
#P connect 178 0 179 0;
#P connect 49 0 50 0;
#P fasten 7 9 49 0 346 328 43 328 43 503 72 503;
#P connect 50 0 51 0;
#P connect 145 0 146 0;
#P fasten 7 33 145 0 658 260 45 260 45 1001 80 1001;
#P connect 146 0 147 0;
#P fasten 7 57 233 0 970 196 46 196 52 1077 110 1077;
#P fasten 7 0 8 0 229 341 111 341;
#P fasten 7 49 201 0 866 217 46 217 46 971 112 971;
#P fasten 7 25 105 0 554 283 45 283 45 840 115 840;
#P fasten 7 17 73 0 450 307 44 307 44 669 118 669;
#P fasten 7 41 169 0 762 240 49 240 49 1159 118 1159;
#P fasten 7 9 41 0 346 330 44 330 44 504 122 504;
#P fasten 7 33 137 0 658 261 45 261 45 1001 130 1001;
#P connect 4 0 3 0;
#P connect 244 0 245 0;
#P connect 245 0 246 0;
#P connect 20 0 21 0;
#P fasten 7 58 244 0 983 194 46 194 46 1076 199 1076;
#P fasten 7 1 20 0 242 342 200 342;
#P connect 21 0 22 0;
#P connect 212 0 213 0;
#P fasten 7 50 212 0 879 215 45 215 45 972 201 972;
#P connect 213 0 214 0;
#P connect 116 0 117 0;
#P fasten 7 26 116 0 567 284 46 284 46 839 204 839;
#P connect 117 0 118 0;
#P connect 84 0 85 0;
#P connect 180 0 181 0;
#P fasten 7 18 84 0 463 306 44 306 44 669 207 669;
#P connect 85 0 86 0;
#P fasten 7 42 180 0 775 240 48 240 48 1160 207 1160;
#P connect 181 0 182 0;
#P connect 52 0 53 0;
#P fasten 7 10 52 0 359 329 45 329 45 504 211 504;
#P connect 53 0 54 0;
#P connect 148 0 149 0;
#P connect 149 0 150 0;
#P fasten 7 34 148 0 671 262 46 262 46 1001 219 1001;
#P connect 16 0 7 0;
#P fasten 7 58 234 0 983 195 46 195 46 1075 252 1075;
#P fasten 7 1 9 0 242 344 253 344;
#P fasten 7 50 202 0 879 216 46 216 46 972 254 972;
#P fasten 7 26 106 0 567 285 47 285 47 840 257 840;
#P fasten 7 18 74 0 463 306 43 306 43 671 260 671;
#P fasten 7 42 170 0 775 241 48 241 48 1161 260 1161;
#P fasten 7 10 42 0 359 329 45 329 45 505 264 505;
#P fasten 7 34 138 0 671 263 47 263 47 1001 272 1001;
#P connect 247 0 248 0;
#P connect 23 0 24 0;
#P fasten 7 59 247 0 996 195 47 195 47 1076 338 1076;
#P connect 248 0 249 0;
#P fasten 7 2 23 0 255 342 339 342;
#P connect 24 0 25 0;
#P connect 215 0 216 0;
#P fasten 7 51 215 0 892 214 45 214 45 973 340 973;
#P connect 216 0 217 0;
#P connect 119 0 120 0;
#P fasten 7 27 119 0 580 285 46 285 46 839 343 839;
#P connect 120 0 121 0;
#P connect 87 0 88 0;
#P connect 183 0 184 0;
#P fasten 7 19 87 0 476 309 44 309 44 671 346 671;
#P connect 88 0 89 0;
#P fasten 7 43 183 0 788 240 48 240 48 1159 346 1159;
#P connect 184 0 185 0;
#P connect 55 0 56 0;
#P fasten 7 11 55 0 372 329 44 329 44 505 350 505;
#P connect 56 0 57 0;
#P connect 151 0 152 0;
#P fasten 7 35 151 0 684 261 46 261 46 1002 358 1002;
#P connect 152 0 153 0;
#P fasten 7 59 235 0 996 196 47 196 47 1075 389 1075;
#P fasten 7 2 10 0 255 342 390 342;
#P fasten 7 51 203 0 892 214 47 214 47 972 391 972;
#P fasten 7 27 107 0 580 287 48 287 48 840 394 840;
#P fasten 7 19 75 0 476 311 45 311 45 670 397 670;
#P fasten 7 43 171 0 788 241 50 241 50 1160 397 1160;
#P fasten 7 11 43 0 372 331 45 331 45 505 401 505;
#P fasten 7 35 139 0 684 262 47 262 47 1003 409 1003;
#P connect 250 0 251 0;
#P connect 251 0 252 0;
#P connect 26 0 27 0;
#P connect 27 0 28 0;
#P fasten 7 60 250 0 1009 196 47 196 47 1077 474 1077;
#P fasten 7 3 26 0 268 343 475 343;
#P connect 218 0 219 0;
#P connect 219 0 220 0;
#P fasten 7 52 218 0 905 214 47 214 47 974 476 974;
#P connect 122 0 123 0;
#P connect 123 0 124 0;
#P fasten 7 28 122 0 593 286 48 286 48 838 479 838;
#P connect 90 0 91 0;
#P connect 91 0 92 0;
#P connect 186 0 187 0;
#P connect 187 0 188 0;
#P fasten 7 20 90 0 489 312 46 312 46 672 482 672;
#P fasten 7 44 186 0 801 239 49 239 49 1162 482 1162;
#P connect 58 0 59 0;
#P fasten 7 12 58 0 385 330 44 330 44 506 486 506;
#P connect 59 0 60 0;
#P connect 154 0 155 0;
#P connect 155 0 156 0;
#P fasten 7 36 154 0 697 264 47 264 47 1003 494 1003;
#P fasten 7 60 236 0 1009 196 47 196 47 1077 526 1077;
#P fasten 7 4 11 0 281 343 527 343;
#P fasten 7 52 204 0 905 215 46 215 46 973 528 973;
#P fasten 7 28 108 0 593 286 47 286 47 840 531 840;
#P fasten 7 20 76 0 489 313 46 313 46 671 534 671;
#P fasten 7 44 172 0 801 240 49 240 49 1161 534 1161;
#P fasten 7 12 44 0 385 332 46 332 46 506 538 506;
#P fasten 7 36 140 0 697 265 48 265 48 1002 546 1002;
#P connect 253 0 254 0;
#P connect 254 0 255 0;
#P connect 29 0 30 0;
#P connect 30 0 31 0;
#P fasten 7 61 253 0 1022 199 46 199 46 1076 610 1076;
#P fasten 7 5 29 0 294 343 611 343;
#P connect 221 0 222 0;
#P connect 222 0 223 0;
#P fasten 7 53 221 0 918 217 46 217 46 974 612 974;
#P connect 125 0 126 0;
#P connect 126 0 127 0;
#P fasten 7 29 125 0 606 285 47 285 47 840 615 840;
#P connect 93 0 94 0;
#P connect 94 0 95 0;
#P connect 189 0 190 0;
#P connect 190 0 191 0;
#P fasten 7 21 93 0 502 313 45 313 45 670 618 670;
#P fasten 7 45 189 0 814 240 49 240 49 1162 618 1162;
#P connect 61 0 62 0;
#P connect 62 0 63 0;
#P fasten 7 13 61 0 398 330 46 330 46 506 622 506;
#P connect 157 0 158 0;
#P fasten 7 37 157 0 710 263 47 263 47 1003 630 1003;
#P connect 158 0 159 0;
#P fasten 7 61 237 0 1022 199 48 199 48 1075 661 1075;
#P fasten 7 5 12 0 294 343 662 343;
#P fasten 7 53 205 0 918 218 46 218 46 975 663 975;
#P fasten 7 29 109 0 606 287 47 287 47 841 666 841;
#P fasten 7 21 77 0 502 314 46 314 46 671 669 671;
#P fasten 7 45 173 0 814 241 49 241 49 1162 669 1162;
#P fasten 7 13 45 0 398 331 46 331 46 506 673 506;
#P fasten 7 37 141 0 710 264 47 264 47 1002 681 1002;
#P connect 256 0 257 0;
#P connect 32 0 33 0;
#P connect 33 0 34 0;
#P fasten 7 62 256 0 1035 199 48 199 48 1076 745 1076;
#P connect 257 0 258 0;
#P fasten 7 6 32 0 307 343 746 343;
#P connect 224 0 225 0;
#P connect 225 0 226 0;
#P fasten 7 54 224 0 931 219 47 219 47 976 747 976;
#P connect 128 0 129 0;
#P connect 129 0 130 0;
#P fasten 7 30 128 0 619 287 47 287 47 842 750 842;
#P connect 96 0 97 0;
#P connect 97 0 98 0;
#P connect 192 0 193 0;
#P connect 193 0 194 0;
#P fasten 7 22 96 0 515 313 45 313 45 671 753 671;
#P fasten 7 46 192 0 827 242 49 242 49 1161 753 1161;
#P connect 64 0 65 0;
#P connect 65 0 66 0;
#P fasten 7 14 64 0 411 332 46 332 46 506 757 506;
#P connect 160 0 161 0;
#P connect 161 0 162 0;
#P fasten 7 38 160 0 723 263 47 263 47 1001 765 1001;
#P fasten 7 62 238 0 1035 200 45 200 45 1076 796 1076;
#P fasten 7 6 13 0 307 344 797 344;
#P fasten 7 54 206 0 931 220 47 220 47 976 798 976;
#P fasten 7 30 110 0 619 288 46 288 46 843 801 843;
#P fasten 7 22 78 0 515 314 46 314 46 671 804 671;
#P fasten 7 46 174 0 827 242 51 242 51 1161 804 1161;
#P fasten 7 14 46 0 411 334 47 334 47 506 808 506;
#P fasten 7 38 142 0 723 264 47 264 47 1002 816 1002;
#P connect 259 0 260 0;
#P connect 260 0 261 0;
#P connect 35 0 36 0;
#P connect 36 0 37 0;
#P fasten 7 63 259 0 1048 200 46 200 46 1078 879 1078;
#P fasten 7 7 35 0 320 344 880 344;
#P connect 227 0 228 0;
#P connect 228 0 229 0;
#P fasten 7 55 227 0 944 218 46 218 46 975 881 975;
#P connect 131 0 132 0;
#P connect 132 0 133 0;
#P fasten 7 31 131 0 632 287 47 287 47 841 884 841;
#P connect 99 0 100 0;
#P connect 100 0 101 0;
#P connect 195 0 196 0;
#P connect 196 0 197 0;
#P fasten 7 23 99 0 528 312 46 312 46 671 887 671;
#P fasten 7 47 195 0 840 241 50 241 50 1159 887 1159;
#P connect 67 0 68 0;
#P connect 68 0 69 0;
#P fasten 7 15 67 0 424 332 47 332 47 505 891 505;
#P connect 163 0 164 0;
#P connect 164 0 165 0;
#P fasten 7 39 163 0 736 262 47 262 47 1003 899 1003;
#P fasten 7 63 239 0 1048 198 48 198 48 1075 931 1075;
#P fasten 7 7 14 0 320 344 932 344;
#P fasten 7 55 207 0 944 220 46 220 46 1005 48 1003 48 973 933 973;
#P fasten 7 31 111 0 632 288 47 288 47 841 936 841;
#P fasten 7 23 79 0 528 313 45 313 45 669 939 669;
#P fasten 7 47 175 0 840 242 49 242 49 1162 939 1162;
#P fasten 7 15 47 0 424 333 48 333 48 505 943 505;
#P fasten 7 39 143 0 736 263 47 263 47 1003 951 1003;
#P connect 262 0 263 0;
#P connect 263 0 264 0;
#P connect 38 0 39 0;
#P connect 39 0 40 0;
#P fasten 7 64 262 0 1061 201 48 201 48 1077 1015 1077;
#P fasten 7 8 38 0 333 344 1016 344;
#P connect 230 0 231 0;
#P connect 231 0 232 0;
#P fasten 7 56 230 0 957 220 48 220 48 975 1017 975;
#P connect 134 0 135 0;
#P connect 135 0 136 0;
#P fasten 7 32 134 0 645 286 46 286 46 841 1020 841;
#P connect 102 0 103 0;
#P connect 103 0 104 0;
#P connect 198 0 199 0;
#P connect 199 0 200 0;
#P fasten 7 24 102 0 541 313 45 313 45 672 1023 672;
#P fasten 7 48 198 0 853 241 49 241 49 1161 1023 1161;
#P connect 70 0 71 0;
#P connect 71 0 72 0;
#P fasten 7 16 70 0 437 332 45 332 45 506 1027 506;
#P connect 166 0 167 0;
#P connect 167 0 168 0;
#P fasten 7 40 166 0 749 262 46 262 46 1002 1035 1002;
#P fasten 7 8 15 0 333 344 1066 344;
#P fasten 7 56 208 0 957 220 48 220 48 973 1067 973;
#P fasten 7 32 112 0 645 287 47 287 47 842 1070 842;
#P connect 7 64 240 0;
#P fasten 7 24 80 0 541 315 46 315 46 670 1073 670;
#P fasten 7 48 176 0 853 244 49 244 49 1163 1073 1163;
#P fasten 7 16 48 0 437 333 46 333 46 504 1077 504;
#P fasten 7 40 144 0 749 263 47 263 47 1001 1085 1001;
#P fasten 270 0 273 0 1221 378 1181 378 1121 158 1153 134;
#P connect 275 0 274 0;
#P connect 273 0 275 0;
#P connect 270 0 269 0;
#P connect 269 0 268 0;
#P fasten 19 0 265 0 61 476 1284 476;
#P fasten 22 0 265 0 200 476 1284 476;
#P fasten 25 0 265 0 339 476 1284 476;
#P fasten 28 0 265 0 474 476 1284 476;
#P fasten 31 0 265 0 610 476 1284 476;
#P fasten 34 0 265 0 745 477 1284 477;
#P fasten 37 0 265 0 879 476 1284 476;
#P fasten 40 0 265 0 1015 478 1284 478;
#P fasten 51 0 265 0 72 647 1250 647 1250 480 1251 479 1284 479;
#P fasten 54 0 265 0 211 648 1250 648 1250 478 1284 478;
#P fasten 57 0 265 0 350 647 1251 647 1251 479 1284 479;
#P fasten 60 0 265 0 486 648 1251 648 1251 480 1284 480;
#P fasten 63 0 265 0 621 649 1252 649 1252 479 1284 479;
#P fasten 66 0 265 0 756 649 1253 649 1253 477 1284 477;
#P fasten 69 0 265 0 890 649 1252 649 1252 480 1284 480;
#P fasten 72 0 265 0 1026 649 1253 649 1253 480 1284 480;
#P fasten 83 0 265 0 68 814 1249 814 1249 479 1284 479;
#P fasten 86 0 265 0 207 814 1250 814 1250 480 1284 480;
#P fasten 89 0 265 0 346 815 1251 815 1251 478 1284 478;
#P fasten 92 0 265 0 481 815 1251 815 1251 480 1284 480;
#P fasten 95 0 265 0 617 816 1251 816 1251 479 1284 479;
#P fasten 98 0 265 0 752 815 1251 815 1251 480 1284 480;
#P fasten 101 0 265 0 886 816 1251 816 1251 479 1284 479;
#P fasten 104 0 265 0 1022 814 1251 814 1251 479 1284 479;
#P fasten 115 0 265 0 65 1003 1249 1003 1249 479 1284 479;
#P fasten 118 0 265 0 204 1003 1251 1003 1251 480 1284 480;
#P fasten 121 0 265 0 343 1003 1252 1003 1252 480 1284 480;
#P fasten 124 0 265 0 478 1004 1252 1004 1252 479 1284 479;
#P fasten 127 0 265 0 614 1005 1252 1005 1252 480 1284 480;
#P fasten 130 0 265 0 749 1004 1252 1004 1252 479 1282 479 1282 496;
#P fasten 133 0 265 0 883 1004 1252 1004 1252 480 1284 480;
#P fasten 136 0 265 0 1019 1005 1251 1005 1251 480 1284 480;
#P fasten 147 0 265 0 82 1161 1251 1161 1251 480 1284 480;
#P fasten 150 0 265 0 218 1162 1252 1162 1252 479 1284 479;
#P fasten 153 0 265 0 358 1163 1253 1163 1253 479 1284 479;
#P fasten 156 0 265 0 493 1162 1253 1162 1253 480 1284 480;
#P fasten 159 0 265 0 632 1161 1253 1161 1253 480 1284 480;
#P fasten 162 0 265 0 764 1163 1254 1163 1254 480 1284 480;
#P fasten 165 0 265 0 898 1163 1253 1163 1253 480 1284 480;
#P fasten 168 0 265 0 1034 1164 1254 1164 1254 479 1284 479;
#P fasten 179 0 265 0 68 1312 1257 1312 1257 480 1284 480;
#P fasten 182 0 265 0 207 1313 1255 1313 1255 481 1284 481;
#P fasten 185 0 265 0 346 1313 1257 1313 1257 480 1284 480;
#P fasten 188 0 265 0 481 1313 1257 1313 1257 480 1284 480;
#P fasten 191 0 265 0 617 1313 1257 1313 1257 478 1284 478;
#P fasten 194 0 265 0 752 1314 1257 1314 1257 480 1284 480;
#P fasten 197 0 265 0 886 1313 1257 1313 1257 480 1284 480;
#P fasten 200 0 265 0 1022 1312 1259 1312 1253 478 1284 478;
#P fasten 211 0 265 0 62 1475 1250 1475 1250 479 1284 479;
#P fasten 214 0 265 0 201 1475 1251 1475 1251 479 1284 479;
#P fasten 217 0 265 0 340 1474 1250 1474 1250 479 1284 479;
#P fasten 220 0 265 0 475 1474 1251 1474 1251 478 1284 478;
#P fasten 223 0 265 0 611 1475 1252 1475 1252 479 1284 479;
#P fasten 226 0 265 0 746 1475 1253 1475 1253 480 1284 480;
#P fasten 229 0 265 0 880 1473 1253 1473 1253 480 1284 480;
#P fasten 232 0 265 0 1016 1474 1253 1474 1253 477 1284 477;
#P fasten 243 0 265 0 60 1599 1257 1599 1257 480 1284 480;
#P fasten 246 0 265 0 198 1599 1257 1599 1257 479 1284 479;
#P fasten 249 0 265 0 338 1598 1257 1598 1257 480 1284 480;
#P fasten 252 0 265 0 473 1599 1258 1599 1258 479 1284 479;
#P fasten 255 0 265 0 609 1599 1258 1599 1258 480 1284 480;
#P fasten 258 0 265 0 745 1599 1259 1599 1259 478 1284 478;
#P fasten 261 0 265 0 878 1599 1260 1599 1260 480 1284 480;
#P fasten 264 0 265 0 1014 1598 1260 1598 1260 480 1284 480;
#P fasten 268 0 265 0 1224 434 1284 434;
#P fasten 267 0 265 0 1225 464 1284 464;
#P connect 265 0 271 0;
#P connect 271 0 272 0;
#P pop;
Labels: code, jitter, max, msp, video